🥷 Clojure Pro Tip 4: Cider ClojureDocs
🥷 Clojure Pro Tip 3: the Thread-As Macro
So you're more than excited to find out how Clojure's thread macros can make code clean and concise, you just love it! But at some point you'll be trapped a bit as the threaded argument's positions are inconsistent using -> or ->>.
Luckily, you can use as-> to place the argument wherever you like:
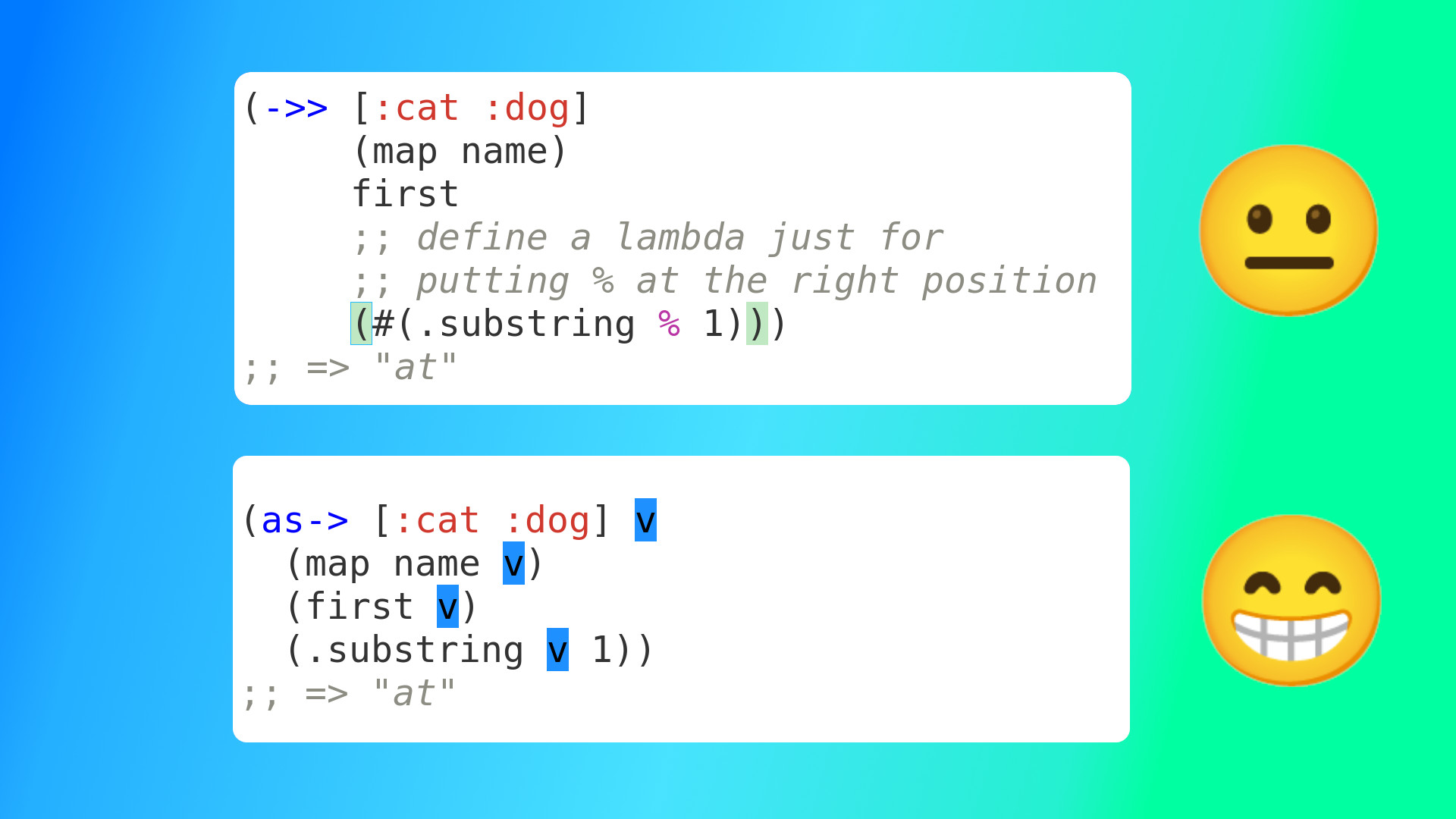
🥷 Clojure Pro Tip 2: group-by
It's amazingly easy to group things in Clojure. The language core provides a group-by function directly. Together with many built-in functions or key functions, it creates a ton of possibilities, and we can also bake our grouping function if none is satisfying.
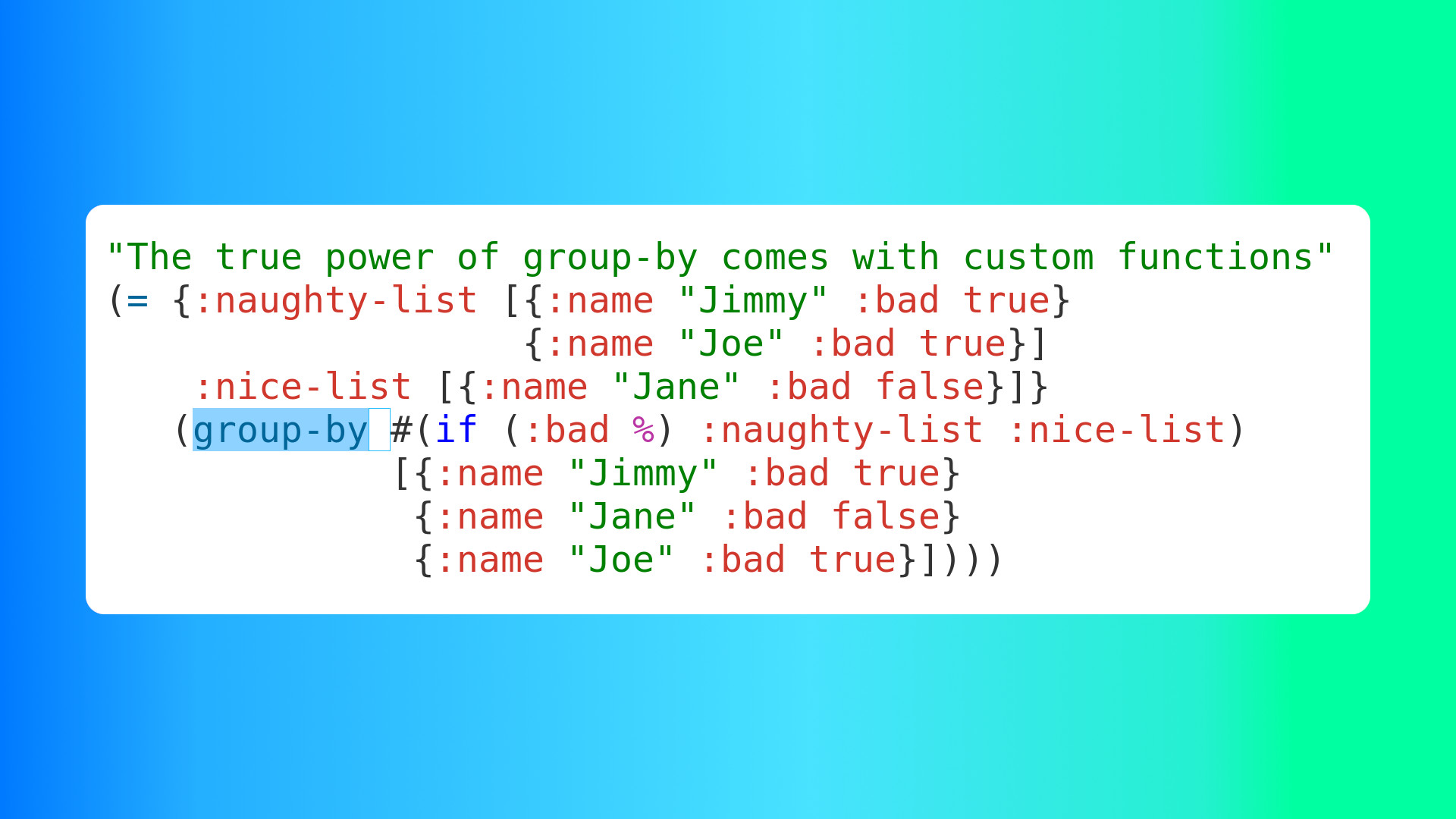
Example from Clojure Koans.
Collecting Events to Google Analytics using ClojureScript
🥷 Clojure Pro Tip 1: the Discard Reader Symbol
In Clojure, we can use comment (aka Rich Comment Blocks) for tests or experiments in development. However, since a comment evaluates to nil, you may run into surprising results or even errors if you misuse it.
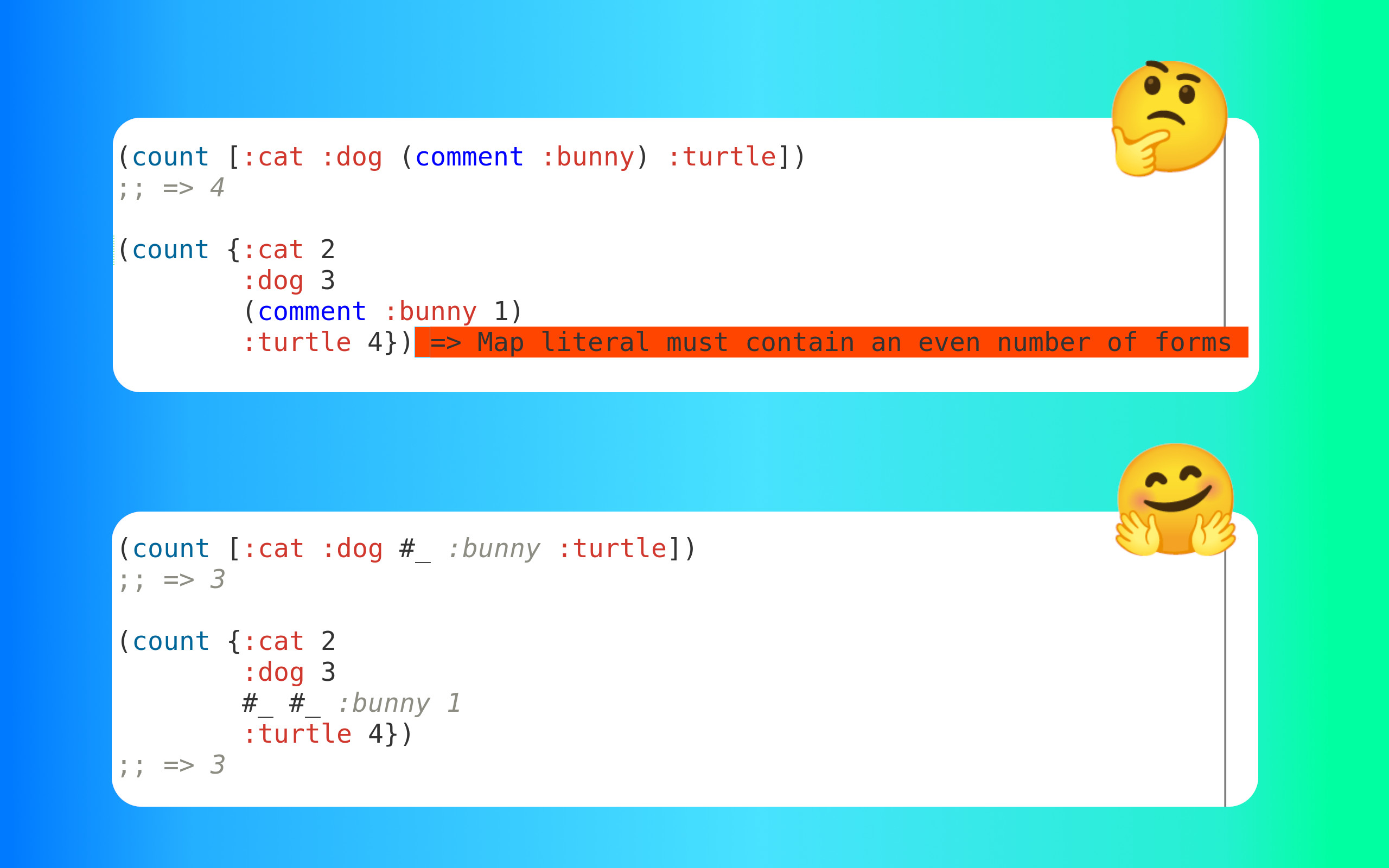
In these scenarios, you may tend to use ;
to comment them out, but a better choice is to use the discard reader symbol #_
.