I'm used to learning by practicing, so when I learned Clojure, I always kept an eye on chances to write code in it.
Scripting is an excellent field to practice, but the experience is not so good. On the one hand, it's too hacky to wrap Clojure code in a shell script with the shell bang. On the other hand, the startup time of JVM is too long to hurt the user experience.
It seems this is a dead end until I found Babashka on Reddit one day, it really blew my mind with its lightning-fast startup speed, and it's also Clojure.
So what can I do with it?
Many websites require us to have a username and a password, and it's risky to have the same or similar passwords for them. But it takes time to come up with passwords for every website. So why not generating random passwords in Babashka?
The requirements for it would be:
- The characters should be random.
- It should be long enough. 12 or 16 is good.
- The password should be a combination of lowercase letters, uppercase letters, numbers, and symbols. Well, this requirement is not met yet.
As for the first one, I learned how to do it in Clojure when I read secrets.clj, java.security
is a good fit for it.
The journey to implement it was fun, as I can write the code bottom-up and top-down, and I can quickly experiment and test the code with REPL.
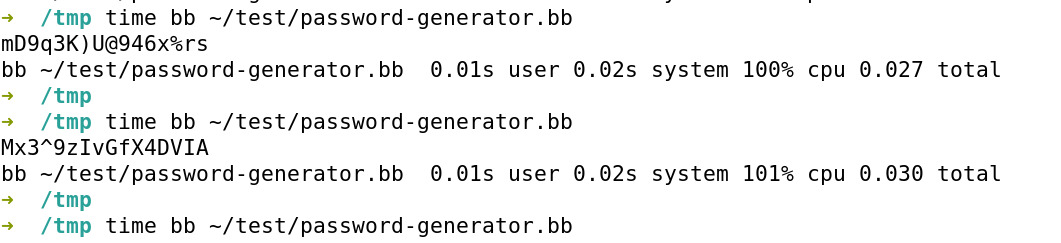
The final code is quite simple:
#!/usr/bin/env bb
(import [java.security SecureRandom])
(def ^:private rng (SecureRandom.))
(let [len 16
passwd (char-array len)
chars (map char (concat (range 48 58) ; 0-9
(range 65 91) ; A-Z
(range 97 123) ; a-z
[\~ \! \@ \# \$ \% \^ \& \* \( \) \- \+ \=]))
nchars (count chars)]
(println (clojure.string/join ""
(mapcat (fn [char]
(str (nth chars (.nextInt rng nchars))))
passwd))))
I make a git repo, babashka-tools, for it at GitHub. I can't wait to have other ideas to write code in Babashka.
Any ideas or suggestions?